![[BOJ5972] 택배 배송(최단경로, 다익스트라)](https://img1.daumcdn.net/thumb/R750x0/?scode=mtistory2&fname=https%3A%2F%2Fblog.kakaocdn.net%2Fdn%2F02Gb5%2FbtssULAcvUJ%2FBleMXHGxSleNvp684uwc8K%2Fimg.png)
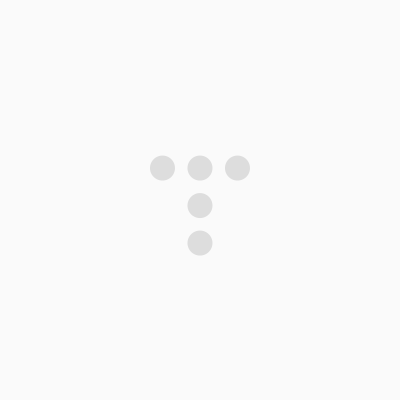
[BOJ5972] 택배 배송(최단경로, 다익스트라)알고리즘/최단 경로2023. 9. 1. 21:35
Table of Contents
반응형
문제 링크
https://www.acmicpc.net/problem/5972
5972번: 택배 배송
농부 현서는 농부 찬홍이에게 택배를 배달해줘야 합니다. 그리고 지금, 갈 준비를 하고 있습니다. 평화롭게 가려면 가는 길에 만나는 모든 소들에게 맛있는 여물을 줘야 합니다. 물론 현서는
www.acmicpc.net
문제 풀이
- 다익스트라 알고리즘 문제
- 1 -> N 노드 까지 최단 거리 구하면 된다
- C_i마리 소 = 간선의 가중치
- 시간 복잡도 : O(ElogV)
- 노드와 간선이 최대 50,000개 있으므로 O(50,000log50,000) = O(50,000 * 약 15.6) , 고로 1초만에 가능
제출 코드
import java.util.*;
import java.io.*;
public class Main {
static List<Info>[] adj;
static int n, m;
static class Info implements Comparable<Info> {
int idx;
int cost;
public Info(int idx, int cost) {
this.idx = idx;
this.cost = cost;
}
@Override
public int compareTo(Info info) {
return cost - info.cost; // 오름차순
}
}
static void input() throws Exception {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
StringTokenizer st = new StringTokenizer(br.readLine());
n = Integer.parseInt(st.nextToken());
m = Integer.parseInt(st.nextToken());
adj = new ArrayList[n + 1];
for(int i = 1; i <= n; i++) adj[i] = new ArrayList<>();
for(int i = 1; i <= m; i++) {
st = new StringTokenizer(br.readLine());
int from = Integer.parseInt(st.nextToken());
int to = Integer.parseInt(st.nextToken());
int cost = Integer.parseInt(st.nextToken());
adj[from].add(new Info(to, cost));
adj[to].add(new Info(from, cost));
}
}
// 1 -> N 까지 최소 비용 구한다
static void dijkstra() {
Queue<Info> que = new PriorityQueue<>();
que.add(new Info(1, 0));
int[] dist = new int[n + 1];
Arrays.fill(dist, Integer.MAX_VALUE);
dist[1] = 0;
int result = Integer.MAX_VALUE;
while(!que.isEmpty()) {
Info cur = que.poll();
if(cur.idx == n) {
result = Math.min(result, cur.cost);
continue;
}
if(dist[cur.idx] < cur.cost) continue;
for(Info next : adj[cur.idx]) {
if(dist[next.idx] > dist[cur.idx] + next.cost) {
dist[next.idx] = dist[cur.idx] + next.cost;
que.add(new Info(next.idx, dist[next.idx]));
}
}
}
System.out.println(result);
}
static void pro() {
dijkstra();
}
public static void main(String[] args) throws Exception {
input();
pro();
}
}
반응형
'알고리즘 > 최단 경로' 카테고리의 다른 글
[BOJ1613] 역사 (플로이드 워셜) (1) | 2023.09.03 |
---|---|
[BOJ1507] 궁금한 민호 (플로이드 워셜) (1) | 2023.09.03 |
[BOJ2660] 회장뽑기 (플로이드 워셜, 최단거리) (0) | 2023.09.02 |
[BOJ1956] 운동 (플로이드 워셜) (0) | 2023.09.02 |
[BOJ1261] 알고스팟 (최단 경로, 다익스트라) (0) | 2023.09.01 |
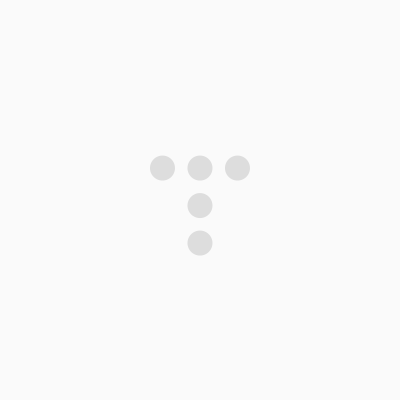
@leejinwoo1126 :: 천천히 하나씩
포스팅이 좋았다면 "좋아요❤️" 또는 "구독👍🏻" 해주세요!