![[BOJ 2096] 내려가기 (Java, DP, 슬라이딩 윈도우)](https://img1.daumcdn.net/thumb/R750x0/?scode=mtistory2&fname=https%3A%2F%2Fblog.kakaocdn.net%2Fdn%2FcRxVOV%2FbtsmPHrnQ4w%2F6RTlKvz0v6Af6X5JzHFsS1%2Fimg.png)
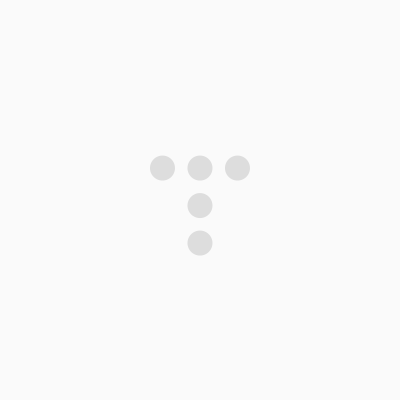
문제 링크
https://www.acmicpc.net/problem/2096
2096번: 내려가기
첫째 줄에 N(1 ≤ N ≤ 100,000)이 주어진다. 다음 N개의 줄에는 숫자가 세 개씩 주어진다. 숫자는 0, 1, 2, 3, 4, 5, 6, 7, 8, 9 중의 하나가 된다.
www.acmicpc.net
문제 풀이
- 시간 복잡도 O(N)
- 메모리 제한 4MB
직접 문제를 풀었을 때 최대 값을 구하는 것은 Bottom-Up방식으로 설명에 따라 그대로 하였기에 구할 수 있었다.
그러나 최소 값의 경우 구할 수 없었고, 기술 블로그를 찾아 본 결과 '메모리 제한', '슬라이딩 윈도우 기법'와 같은 파악하지 못 한 부분에 대해서도 확인할 수 있었다. (최소값의 경우 반대로 선택 가능 범위 내에 최소 값을 더해 갱신해주는 형태였다 하하..)
초기
1 | 2 | 3 |
4 | 5 | 6 |
4 | 9 | 0 |
최대
행을 내려갈 때 마다 이전 행에 선택 가능 범위 내에 최대값을 구해서 합한 결과를 구함
1 | 2 | 3 |
6 | 8 | 9 |
14 | 18 | 9 |
최소
행을 내려갈 때 마다 이전 행에 선택 범위 내에 최소값을 구해서 합한 결과를 구함
1 | 2 | 3 |
5 | 6 | 8 |
9 | 14 | 6 |
참고
https://www.youtube.com/watch?v=uH9VJRIpIDY
https://steady-coding.tistory.com/154
[BOJ] 백준 2096번 : 내려가기 (JAVA)
문제 N줄에 0 이상 9 이하의 숫자가 세 개씩 적혀 있다. 내려가기 게임을 하고 있는데, 이 게임은 첫 줄에서 시작해서 마지막 줄에서 끝나게 되는 놀이이다. 먼저 처음에 적혀 있는 세 개의 숫자
steady-coding.tistory.com
제출 코드
import java.util.*;
import java.io.*;
public class Main {
static StringBuilder sb = new StringBuilder();
static InputProcessor inputProcessor = new InputProcessor();
static int N, MAX_VALUE, MIN_VALUE;
static int[][] DATA;
static int[][] MAX_DP, MIN_DP;
public static void main(String[] args) throws IOException {
input();
pro();
output();
}
private static void input() {
N = inputProcessor.nextInt();
DATA = new int[N + 1][3];
for(int i = 1; i <= N; i++) {
for(int j = 0; j < 3; j++) {
DATA[i][j] = inputProcessor.nextInt();
}
}
// 초기화
MAX_DP = new int[N + 1][3];
MAX_DP[1][0] = DATA[1][0];
MAX_DP[1][1] = DATA[1][1];
MAX_DP[1][2] = DATA[1][2];
MIN_DP = new int[N + 1][3];
MIN_DP[1][0] = DATA[1][0];
MIN_DP[1][1] = DATA[1][1];
MIN_DP[1][2] = DATA[1][2];
MAX_VALUE = 0;
MIN_VALUE = Integer.MAX_VALUE;
}
private static void pro() {
bottomUp();
}
private static void bottomUp() {
for (int i = 2; i <= N; i++) {
// 최대값
MAX_DP[i][0] = Math.max(MAX_DP[i - 1][0], MAX_DP[i - 1][1]) + DATA[i][0];
MAX_DP[i][1] = Math.max(MAX_DP[i - 1][0], Math.max(MAX_DP[i - 1][1], MAX_DP[i - 1][2])) + DATA[i][1];
MAX_DP[i][2] = Math.max(MAX_DP[i - 1][1], MAX_DP[i - 1][2]) + DATA[i][2];
//최소값
MIN_DP[i][0] = Math.min(MIN_DP[i - 1][0], MIN_DP[i - 1][1]) + DATA[i][0];
MIN_DP[i][1] = Math.min(MIN_DP[i - 1][0], Math.min(MIN_DP[i - 1][1], MIN_DP[i - 1][2])) + DATA[i][1];
MIN_DP[i][2] = Math.min(MIN_DP[i - 1][1], MIN_DP[i - 1][2]) + DATA[i][2];
}
for (int i = 0; i <= 2; i++) {
MIN_VALUE = Math.min(MIN_VALUE, MIN_DP[N][i]);
MAX_VALUE = Math.max(MAX_VALUE, MAX_DP[N][i]);
}
sb.append(MAX_VALUE).append(" ").append(MIN_VALUE);
}
private static void output() throws IOException {
BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(System.out));
bw.write(sb.toString());
bw.flush();
bw.close();
}
private static class InputProcessor {
BufferedReader br;
StringTokenizer st;
public InputProcessor() {
this.br = new BufferedReader(new InputStreamReader(System.in));
}
public String next() {
while(st == null || !st.hasMoreElements()) {
try {
st = new StringTokenizer(br.readLine());
} catch (IOException e) {
throw new RuntimeException(e);
}
}
return st.nextToken();
}
public String nextLine() {
String input = "";
try {
input = br.readLine();
} catch (IOException e) {
throw new RuntimeException(e);
}
return input;
}
public int nextInt() {
return Integer.parseInt(next());
}
public long nextLong() {
return Long.parseLong(next());
}
}
}
'알고리즘 > 동적 프로그래밍' 카테고리의 다른 글
[BOJ 5557] 1학년 (Java, DP) (0) | 2023.07.10 |
---|---|
[BOJ 2193] 이친수 (Java, DP) (0) | 2023.07.10 |
[BOJ 1562] 계단수 (Java, DP, Bit Masking) (0) | 2023.07.04 |
[BOJ 2688] 줄어들지 않아 (Java, DP) (0) | 2023.07.04 |
[BOJ 9465] 스티커 (Java, DP) (0) | 2023.07.04 |
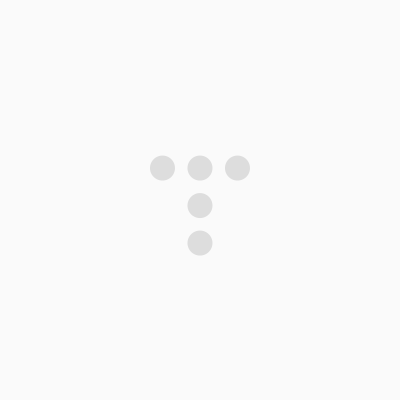
포스팅이 좋았다면 "좋아요❤️" 또는 "구독👍🏻" 해주세요!