![[BOJ 10844] 쉬운 계단 수 (Java, DP)](https://img1.daumcdn.net/thumb/R750x0/?scode=mtistory2&fname=https%3A%2F%2Fblog.kakaocdn.net%2Fdn%2FAzWIr%2Fbtsnfs0kRXv%2FzKZULqvrRqwDFgmDdb3IVk%2Fimg.png)
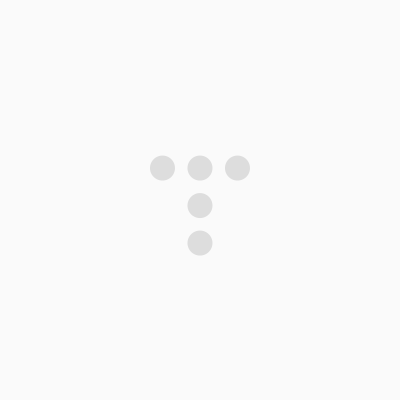
[BOJ 10844] 쉬운 계단 수 (Java, DP)알고리즘/동적 프로그래밍2023. 6. 30. 21:39
Table of Contents
반응형
문제 링크
https://www.acmicpc.net/problem/10844
10844번: 쉬운 계단 수
첫째 줄에 정답을 1,000,000,000으로 나눈 나머지를 출력한다.
www.acmicpc.net
문제 풀이
- 시간 복잡도 : O(N * 10) = O(N)
- MOD를 나누지 않으면 터지는 이유 확인 필요
DP[i][j] = i 는 자리수 이고, j는 j로 끝나는 수를 뜻함
i\j | 0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 |
1 | 0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 |
2 | 10 | 21 | 12, 32 | 23, 43 | 34, 54 | 45, 65 | 56, 76 | 67, 87 | 78, 98 | 89 |
제출 코드
1) Bottom-Up 방식
import java.util.*;
import java.io.*;
public class Main {
static int N;
static int[][] DP;
static int MOD = 1000000000;
static void input() throws Exception {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
N = Integer.parseInt(br.readLine());
DP = new int[N + 1][10];
for(int i = 1; i <=9; i++) {
DP[1][i] = 1;
}
}
static void pro() {
for(int i = 2; i <= N; i++) {
for(int j = 0; j <= 9; j++) {
DP[i][j] = 0;
if(j > 0) DP[i][j] += DP[i - 1][j - 1];
if(j < 9) DP[i][j] += DP[i - 1][j + 1];
DP[i][j] %= MOD;
}
}
int result = 0;
for(int v : DP[N]) {
result += v;
result %= MOD;
}
System.out.println(result);
}
public static void main(String[] args) throws Exception {
input();
pro();
}
}
2) Top-Down
import java.util.*;
import java.io.*;
public class Main {
static int N;
static Long[][] _DP;
static int MOD = 1000000000;
static void input() throws Exception {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
N = Integer.parseInt(br.readLine());
_DP = new Long[N + 1][10];
for(int i = 1; i <=9; i++) {
_DP[1][i] = 1L;
}
_DP[1][0] = 0L;
}
static void proByTopDown() {
long result = 0L;
for(int i = 0; i <= 9; i++) {
result += topDown(N, i);
result %= MOD;
}
System.out.println(result);
}
static long topDown(int depth, int idx) {
if(depth == 1){
return _DP[depth][idx];
}
if(_DP[depth][idx] == null) {
if (idx == 0) {
_DP[depth][idx] = topDown(depth - 1, idx + 1);
} else if (idx == 9) {
_DP[depth][idx] = topDown(depth - 1, idx - 1);
} else {
_DP[depth][idx] = topDown(depth - 1, idx - 1) + topDown(depth - 1, idx + 1);
}
}
return _DP[depth][idx] %= MOD;
}
public static void main(String[] args) throws Exception {
input();
proByTopDown();
}
}
반응형
'알고리즘 > 동적 프로그래밍' 카테고리의 다른 글
[BOJ 14002] 가장 긴 증가하는 부분 수열 (Java, DP, LIS) (0) | 2023.06.30 |
---|---|
[BOJ 18353] 병사 배치하기 (Java, DP, LIS) (0) | 2023.06.30 |
[BOJ 1463] 1로 만들기 (Java, DP) (0) | 2023.06.30 |
[BOJ 2156] 포도주 시식(Java, DP) (0) | 2023.06.30 |
[BOJ 2302] 극장 좌석 (Java, DP) (0) | 2023.06.29 |
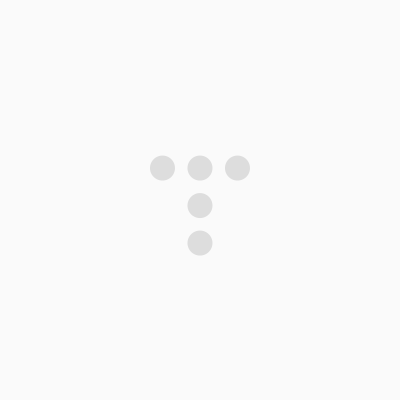
@leejinwoo1126 :: 천천히 하나씩
포스팅이 좋았다면 "좋아요❤️" 또는 "구독👍🏻" 해주세요!